汤浩主页
很多年前大学甚至高中的时候我就开始想有自己的个人主页。事实是这些年来确实也都有。但是由于很多原因都没有坚持写。对于我而言,如果不记笔记,知识就没有内化,没有充分理解和掌握。我大学毕业的时候有一个小遗憾。当时从比利时搬家去上海,为了轻车简从,把大学期间打印的讲义和笔记都扔掉了,堆砌起来可能有将近一立方米。后来有些知识想捡起来继续看一下,也找不着了。只能作为经验教训了。
一直没有坚持写的另一个原因是,以前写的东西都零散的散落在互联网的各个角落。从 Blogger 到 msn space 再到简书。在互联网行业,没有任何平台是永生的。为什么要为了这些每几年就会消失的平台去耗费时间精力去写呢?
这页是用 markdown 写的(严格说其实 markdown 的语法基本都没用到)。它最大的意义在于,这页存在 Github 上。如果 Github 要关闭了,我还可以转到下一个版本管理平台上。这一点很重要,因为如果用其他发布文章的平台,文字和渲染通常是混在一起的。如果搞迁移,排版会乱的话相当于之前写的都白费了。但是因为这页是用 markdown 写的,可以一直用 github 这类版本管理工具保存。markdown 文件可以用很多不同的工具比如 11ty,Hexo 或者 Jekyll等渲染。
长久以来我都想把写的东西弄到版本管理里去。直到现在才有时间去梳理这些一直想做却因为时间和借口没有做的事情。
这个站目前用静态页面的解决方案 11ty 来渲染。不过有些细节还没调好。比如刷题笔记因为题目比较多,需要一个目录在左边。导致那页现在没法把左边的菜单栏加进去。之前用 Hexo 渲染的主页由于刷题笔记页在移动端浏览器渲染会崩溃,被迫升级了一下 Hexo 和 Next,然后样式就调不回去了。因为想要较深度的订制,我一直都想用静态页面解决方案而不是内容管理(CMS)类的工具如 WordPress 来建个人站。最近机遇巧合发现 11ty,再加上 Hexo 和 Next 已经调不好渲染的样式了,就迁移到 11ty了。
再之前用过 Octopress,主题 greyshade 最令我满意。截图如下:
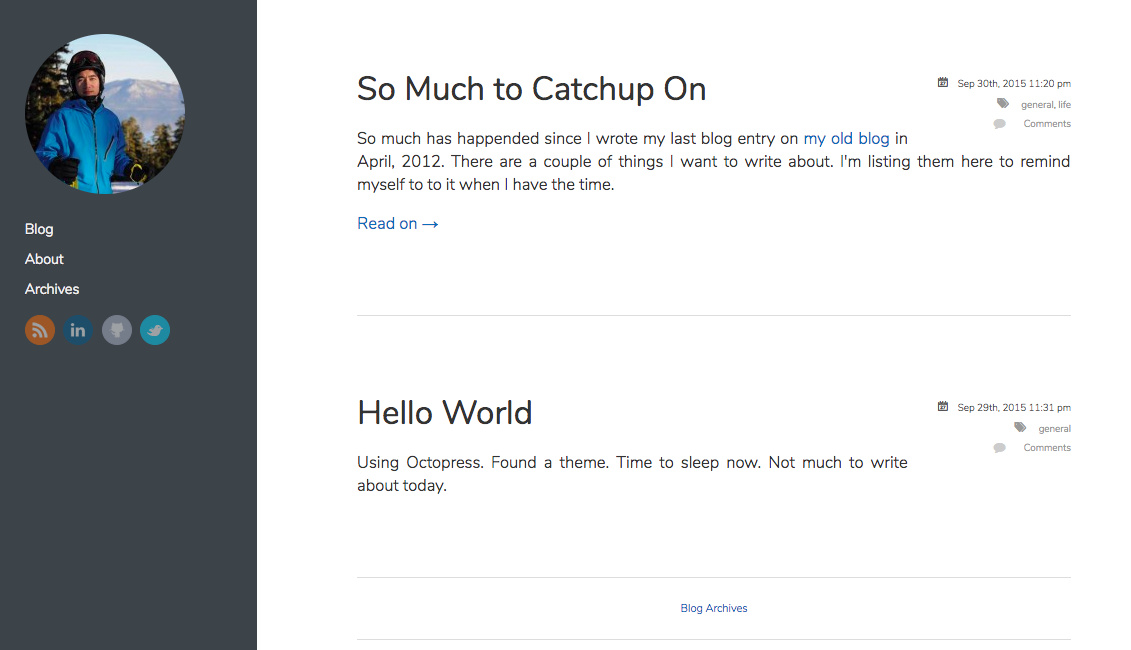
Octopress 是基于 Jekyll 开发的,Jekyll 是用 Ruby On Rails 写的,由于我不熟悉 Ruby,配置实在是搞不定。几年前 Octopress 项目页停止维护了。在网上寻找替代方案的时候发现 Github 上 greyshade 的例子,基本上都是用的 Hexo 和 Next。就用了几年 Hexo 和 Next。
最后一次编辑于 2024-06-15